Hangman Python Code
Creating a Hangman game in Python is a fun and educational project. Here’s a simplified version of the code to get you started:
import random
# List of words for the game
words = [“python”, “hangman”, “programming”, “code”, “computer”, “challenge”]
# Select a random word from the list
word_to_guess = random.choice(words)
# Initialize variables
guesses = []
max_attempts = 6
attempts = 0
# Main game loop
while True:
# Display the current progress
display_word = “”
for letter in word_to_guess:
if letter in guesses:
display_word += letter
else:
display_word += “_”
print(“Word to guess: ” + display_word)
Creating a Hangman Game in Python:
If you’re a fan of classic word-guessing games and want to hone your Python programming skills, creating a Hangman game is a fun and educational project to undertake. Hangman is a game that involves guessing a secret word letter by letter while avoiding a series of incorrect guesses that lead to the drawing of a hanging man.
In this step-by-step guide, we will explore the process of creating a Hangman game using Python. This project will cover essential Python programming concepts and provide you with valuable insights into game development.
Getting Started
Before diving into the code, you need to have Python installed on your computer. If you haven’t already installed it, visit the official Python website. (https://www.python.org/downloads/) and download the latest version for your operating system. For more information visit our website techfuses.com
Additionally, you may choose to work in a code editor or integrated development environment (IDE) of your preference. Popular options include PyCharm, Visual Studio Code, and Jupyter Notebook. Make sure your chosen environment is set up and ready for Python development.
The Hangman Game Overview
Before delving into the coding aspect, let’s understand the rules and objectives of the Hangman game:
Objective: Guess the secret word correctly by suggesting letters, one at a time.
Rules:
The guessing player suggests one letter at a time.
If the suggested letter is in the word, it replaces the corresponding dashes.
If the suggested letter is not in the word, a part of the hangman is drawn.
The game continues until the word is guessed correctly or the hangman is fully drawn (indicating a loss).
Required Python Libraries
In building our Hangman game, we will utilise several built-in Python libraries:
Random: To select a random word for the game.
String: For working with strings and characters efficiently.
Collections: Useful for creating data structures to track game progress.
Time: For adding delays or timing elements to the game.
These libraries will be crucial for different aspects of the game’s development.
Designing the User Interface
A key part of creating an engaging Hangman game is designing a user-friendly interface. In our case, we’ll focus on a console-based interface, but you can extend this to a graphical user interface (GUI) if desired.
ASCII Art for the Hangman: Adding visual elements, such as ASCII art for the hangman’s gallows and the hanging figure, enhances the game’s visual appeal. There are various ASCII art designs available online, or you can create your own.
User-Friendly Console Interface: The console interface should provide clear instructions and feedback to the player. Consider implementing elements like a welcoming message, a display of the hangman ASCII art, the current word with dashes and correctly guessed letters, prompts for player input, feedback on correctness of guesses, a display of incorrect guesses, and messages indicating a win or loss.
Creating a well-designed interface enhances the player’s experience and makes the game more enjoyable.
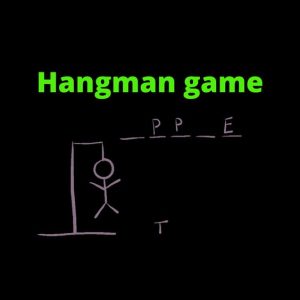
Building the Core Game Logic
With the user interface in mind, you can start working on the core game logic:
Selecting a Random Word: Utilise Python’s random library to select a random word for the game. You can create a list of words from which to choose.
Displaying Word Placeholders: Use strings and characters to display placeholders for the letters in the secret word. Dashes are commonly used as placeholders.
Handling User Input: Implement user input mechanisms to receive letter guesses.
Checking and Updating the Word Display: Compare the guessed letter with the secret word and update the display accordingly.
Implementing Game Mechanics
In addition to the core game logic, you’ll need to implement game mechanics to keep track of progress and determine win or loss conditions:
Keeping Track of Incorrect Guesses: Create a mechanism to count and display the number of incorrect guesses.
Drawing the Hangman: Develop logic to draw the hangman piece by piece as incorrect guesses accumulate.
Determining Win or Loss Conditions: Implement checks to determine if the player has won or lost the game.
Handling Game Over Scenarios: Display appropriate messages and allow the player to restart the game after a win or loss.
Enhancing the Hangman Game
Once you have a working version of Hangman, you can enhance it with advanced features:
Adding Difficulty Levels: Incorporate different difficulty levels with varying word lengths or limited incorrect guesses.
Incorporating a Word Database: Expand the word selection by using a database or API to fetch words.
Implementing a Scoring System: Assign scores based on player performance.
Creating a Multiplayer Version: Extend the game to allow multiplayer interactions.
Testing and Debugging Your Hangman Code
Testing is a critical part of software development. To ensure your Hangman game is robust and free of errors, consider the following:
Unit Testing Strategies: Create unit tests to validate individual functions and components of your code.
Debugging Common Issues: Be prepared to troubleshoot common issues such as incorrect word selection or display problems.
Error Handling and Edge Cases: Implement error handling for unexpected situations to provide a smooth gaming experience.
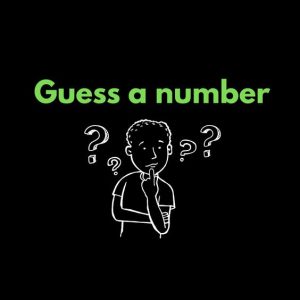
Conclusion
Creating a Hangman game in Python is not only an enjoyable programming project but also a valuable learning experience. Through this endeavor, you’ll gain proficiency in Python, improve your problem-solving skills, and enhance your understanding of game development concepts.
We’ve covered the essential steps to get you started on your Hangman project, from setting up your Python environment to designing the user interface, building the core game logic, and implementing advanced features. With dedication and creativity, you can craft a Hangman game that provides hours of entertainment and serves as a testament to your programming skills.
FAQs
What is Hangman Python Code?
Hangman Python code is a program written in Python that simulates the classic word-guessing game, Hangman. Players guess letters to uncover a hidden word, while the code handles game logic.
Is Python the best language for creating a Hangman game?
Python is an excellent choice for creating a Hangman game due to its simplicity and readability. However, you can implement Hangman in other programming languages as well.
Do I need to be an experienced programmer to create Hangman in Python?
No, you can create a basic Hangman game with just a beginner’s understanding of Python. As you gain experience, you can enhance and customize your game further.
Can I add additional features to my Hangman game?
Absolutely! You can extend your Hangman game by adding features like difficulty levels, and multiplayer support, or integrating it with a GUI for a more interactive experience.
Are there resources available to learn Hangman Python Code?
Yes, there are numerous tutorials, online courses, and code examples available to help you learn and master Hangman Python code. Online Python communities can also provide valuable support.